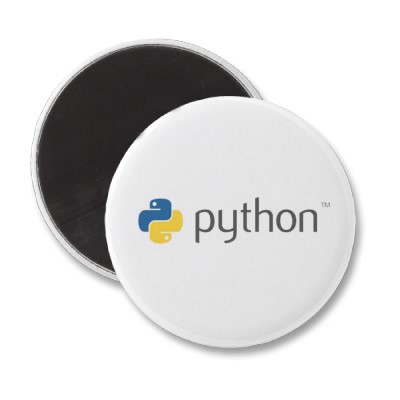
Python Thread Practice
Hey friends, okay so this little tutorial is all about your first workings with threads in python.
What?
Threads are common in many lower level programming languages, they come in different forms / difficulties and handle with different effects because of these. A thread inside your program is a separate CPU instance that is used to generally execute a “task” concurrently whilst you process a different workload.
Why?
Using threads can be equally good or equally dangerous, threads should only be used when you have a clear definitive idea of why you want to use them. I see people all the time say “I use threads in this because I like to split things up, it makes it faster” — However many of these people fail to understand the implications of using threads, such as the debugging and careful strategy for data handling that needs to be put into these to ensure you have not only a stable system but correct data handling technique to ensure you don’t get unexpected output or data corruption / loss.
I tend to use threads when I want to get another stack to process a large amount of data, I usually implement this choice when I am waiting / polling a main data source and using kind of a queue -> processor style setup.
However there is many uses for threads, but please – take caution and read heavily into threading before you attempt it on production software 🙂
How?
So lets implement a basic threaded program, we shall call it the “threaded_HelloWorld.py” (seems fitting doesn’t it?)
1) Open a new document in your editor of choice (mines “vim”)
# vim threaded_HelloWorld.py
2) Make sure you put at the head of your document the python environment execution path:
#!/usr/bin/python2.6
3) import the threading and time modules:
import threading, time;
4) Now lets define a function that will serve as your thread instance, we will call it “myThread”:
def myThread(threadID): print "Hello from thread %d " % threadID; time.sleep(10); //Sleep for ten seconds print "Bye from thread %d " % threadID;
5) now we put our main program execution
threads = [1, 2, 3, 4, 5]; for threadID in threads: th = threading.Thread(target=myThread, args=(threadID)); th.start();
Now save this document and run the following to ensure it’s an executable:
# chmod +x threaded_HelloWorld.py
Now lets run the script:
# ./threaded_HelloWorld.py
It should output something like:
Hello from thread 1
Hello from thread 2
Hello from thread 3
Hello from thread 4
Hello from thread 5
Bye from thread 1
Bye from thread 2
Bye from thread 3
Bye from thread 4
Bye from thread 5
As you can see, the threads start and end!
Enjoy!
Karl.
Hosting Options & Info | VPS | Web Solutions & Services |
---|---|---|