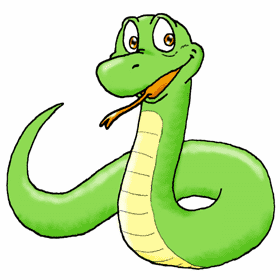
Python: Make Tables That Look Readable in the Command Line
Hey coders, long time no speak?
Today I want to share with you a quick little tutorial in making nice looking tables (pretty much the same style of table that you see in a MySQL cli output).
What I am trying to achieve here is this:
<a href="https://www.crucial.com.au/blog/2012/08/15/python-make-tables-that-look-readable-in-the-command-line/prettytable/" rel="attachment wp-att-2196"><img class="alignleft size-full wp-image-2196" title="prettyTable" src="https://www.crucial.com.au/blog/wp-content/uploads/2012/08/prettyTable.jpg" alt="" width="415" height="194" /></a>
To implement this we use the plugin “PrettyTable”, which is available from: http://code.google.com/p/prettytable/
Installation:
Just for reference, I am using Python2.6 on CentOS.
To install, download the latest copy from code.google ^ Link is above.
Example:
# cd ~/; wget http://prettytable.googlecode.com/files/prettytable-0.6.tar.gz
Then untar and move into the directory:
# tar zxvf prettytable-0.6.tar.gz; cd prettytable-0.6
Install that plugin!
# python2.6 setup.py install
If that command throws up an error like :
Traceback (most recent call last):
File “setup.py”, line 2, in <module>
from setuptools import setup
ImportError: No module named setuptools
To overcome this you just need to install setuptools, like so:
# yum install python26-setuptools
Now run that setup command again (python2.6 setup.py install) and you should be ready to pretty your outputs.
Using the plugin:
Okay so to use this plugin in it’s basic form is pretty simple, go create a python script:
# vim myFancyScript.py
Now because you compiled and installed with Python2.6 you need to ensure you bashenv interpreter is set correctly! – At the top of the file we have open place this:
#!/usr/bin/python26
Note: ensure you have the # (pound) in this line.
Now you’re ready to import the module and do fancy stuff, import it as shown by adding the following lines to your file:
from prettytable import PrettyTable
You now have prettyTables ready to use, in the file lets create a table and add some data, first we declare pretty table and put the column headings in, like shown:
x = PrettyTable([“City name”, “Area”, “Population”, “Annual Rainfall”])
Now you can add a few rows and make this script do something, add a few rows like shown:
x.add_row([“Adelaide”,1295, 1158259, 600.5])
x.add_row([“Brisbane”,5905, 1857594, 1146.4])
x.add_row([“Darwin”, 112, 120900, 1714.7])
x.add_row([“Hobart”, 1357, 205556, 619.5])
x.add_row([“Sydney”, 2058, 4336374, 1214.8])
x.add_row([“Melbourne”, 1566, 3806092, 646.9])
x.add_row([“Perth”, 5386, 1554769, 869.4])
You can now print this to the console like so:
print x;
You now have a a working script, lets save it and run it, do the following:
1) save the file in vim, esc + : + wq + return/enter
Run this:
# chmod +x myFancyScript.py
# ./myFancyScript.py
Now it should output the following:
<a href="https://www.crucial.com.au/blog/2012/08/15/python-make-tables-that-look-readable-in-the-command-line/prettytable/" rel="attachment wp-att-2196"><img class="alignleft size-full wp-image-2196" title="prettyTable" src="https://www.crucial.com.au/blog/wp-content/uploads/2012/08/prettyTable.jpg" alt="" width="415" height="194" /></a>
Enjoy and happy coding!
–Karl.
Hosting Options & Info | VPS | Web Solutions & Services |
---|---|---|